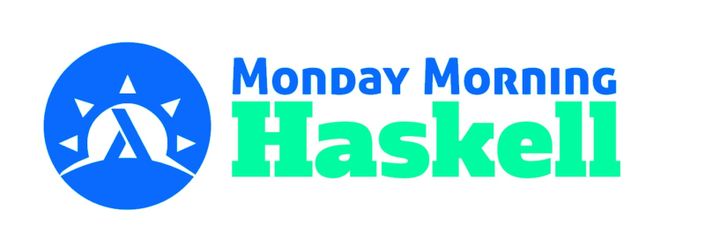
Solve.hs
Learn Problem Solving in Haskell!
Solve.hs
Do you feel like you're "fluent" in Haskell yet? If you're anything like I was a few years ago, you may have been trying out Haskell on the side for quite a while, but you haven't really gotten the hang of it yet. It still feels like a foreign language.
Whenever you want to write some code, it feels like you have to first think of that code in your head as if it were in a more common language like C++ or Python. You then have to translate that idea into Haskell, and it feels like a very arduous process each time. As a result, even relatively simple tasks seem to take quite a while for you to write, and so learning Haskell feels like a tiring task. You might be asking some of these seemingly simple questions:
- How do I write for-loop code in Haskell?
- Why is recursion so important in Haskell?
- What convenience functions exist for list manipulation?
- How do data structures work if Haskell is immutable?
Solve.hs is designed to improve your fluency in Haskell by teaching you the fundamental patterns of problem solving in this fascinating language. If you want to learn more about what's in this course, keep reading!
Course Content and Outline
The first core component of this course is video lectures. You'll watch videos with accompanying slides while learning about a new set of essential ideas. Then, you'll try the ideas for yourself with our in-depth programming exercises. These exercises will give you the chance to try the ideas for yourself in your Haskell environment, with a battery of unit tests that will provide a quick feedback loop on whether or not you are able to implement the concepts.
Module 1: Lists and Loop Patterns
For now, the course has 2 modules (2 additional modules will be released in 2024). The first module covers Haskell's List type in great detail, showing the variety of different API functions you can use with it, as well as the patterns you can apply with the type. You'll get the chance to essentially rebuild the type from scratch yourself, including much of its core and even auxiliary API. This will help you practice the many list patterns out there, and give you a greater familiarity with the "shortcuts" that are available in the API!
Part of this module's focus is to reconstruct our most basic understanding of Haskell's list patterns in explicit terms. So while a fair amount of the material looks "simple" on the surface, this reconstruction will help us draw parallels to constructs in other languages (especially 'for' and 'while' loops). So it's worthwhile even if you've been working with Haskell for a while!
Module 2: Data Structures
Once we've covered lists, we'll move on to Module 2. There, we will survey the many other core structures that allow us to solve problems more efficiently. We'll learn about how Haskell's immutability causes some significant differences in how many of these structures are implemented! We'll learn what the performance implications of this are, and when this matters.
You'll also get the chance to implement a couple different structures from scratch, similar to the Module 1 work with lists (though we'll focus on core APIs here instead of auxiliary helpers).
For more details, see the full outline and FAQ below!
Your Instructor
James is a Software Engineer living in Atlanta, GA. He first discovered Haskell in college, but began using it consistently since 2015. His Haskell experience blends multiple side projects as well as professional work. In late 2016, he began the Monday Morning Haskell blog, where he publishes new Haskell content every week.
Course Curriculum
-
StartLecture 1: Introduction & Pattern Matching (15:43)
-
StartLecture 2: Recursion Basics (9:05)
-
StartLecture 3: Recursion with Accumulation (6:51)
-
StartLecture 4: Tail Recursion (7:32)
-
StartLecture 5: List Accumulation (5:46)
-
StartLecture 6: Folds (10:56)
-
StartLecture 7: Higher Order Function Patterns (8:13)
-
StartLecture 8: Set Operations (5:31)
-
StartLecture 9: Monadic Operations (9:32)
-
StartLecture 10: State Evolution Patterns (12:07)
-
StartLecture 11: Module Review & Practice Problems (2:48)
-
StartLecture 1: Sets & Binary Search Trees (19:58)
-
StartLecture 2: Balancing Binary Search Trees (9:40)
-
StartLecture 3: Maps and the Common API (13:12)
-
StartLecture 4: Hash Sets & Hash Maps (13:09)
-
StartLecture 5: Vectors & Arrays (14:33)
-
StartLecture 6: Mutable Arrays & Hash Tables (14:05)
-
StartLecture 7: Queues & Sequences (11:58)
-
StartLecture 8: Heaps & Greedy Algorithms (19:11)
-
StartLecture 9: Bit Sets & Binary Manipulation (12:19)
-
StartLecture 10: JSON Types & Module Review (4:05)
-
StartLecture 1: Mutual Recursion (10:40)
-
StartLecture 2: Use It or Lose It (11:23)
-
StartLecture 3: Memoization (12:57)
-
StartLecture 4: Dynamic Programming (18:05)
-
StartLecture 5: Search Problems & Depth First Search (18:01)
-
StartLecture 6: Breadth First Search (7:41)
-
StartLecture 7: Weighted Search & Dijkstra's Algorithm (20:26)
-
StartLecture 8: Heuristics and the A* Algorithm (18:56)
-
StartLecture 9: Floyd Warshall Algorithm (15:36)
-
StartLecture 10: Max-Flow & Min-Cuts (11:41)
-
StartLecture 11: Cycle Detection (12:20)