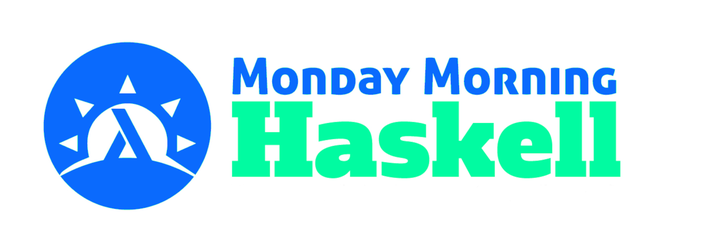
Haskell from Scratch
A Beginner's Guide to Haskell
Haskell From Scratch
If you're discovering this course, you've probably read quite a bit of Haskell. But perhaps you've never written any of it yourself. Or maybe you tried some tutorial, and got a few lines to work. But you don't know how you would actually build a project in Haskell.
If you think it's time to change that, keep reading!
Our Haskell From Scratch Course is an awesome tool for newcomers to Haskell, focusing on all the questions you'll have when you're just starting out:
- How do I build and run Haskell code?
- How does Haskell's type system work?
- What is a monad?
- How is problem solving different in Haskell?
You'll learn about all of this and more in Haskell From Scratch.
Course Content and Outline
The course content consists of 3 elements:
- Video Lectures
- Programming Exercises
- Final Project
In video lectures, your instructor will introduce the core concepts of the lesson, including an in-depth breakdown of the code you need to implement the feature.
You'll then get the chance to try the concepts for yourself with our detailed programming exercises. These exercises are the most important part of the course! By writing the code and applying the concepts yourself, you'll build up the confidence to use these ideas in your own projects.
After the 7 learning modules, you then get the chance to work on a "Final Project" that will test the skills you've learned about implementing and running Haskell code.
Module 1: Basics
In module 1, you'll learn about the fundamental building blocks of Haskell's syntax. You'll also learn the important commands of navigating a Haskell project, like building code, running tests, and experimenting with code with the GHC Interpreter.
Module 2: Data Types
Making your own data types requires a lot of boilerplate in some languages (think about C++ and constructors, destructors, assignment operators, etc.) In Haskell, data types are a lot simpler! We'll go over the various options you have in defining types, as well as the idea of typeclasses and how they enable inheritance.
Module 3: Lists and Recursion
Lists are the simplest way to structure data in Haskell. In this module, we'll learn a lot of interesting ways to construct and manage lists in Haskell. Since lists are a fundamentally recursive structure, we'll also start to learn about recursion as the root of functional problem solving techniques.
Module 4: IO Actions
In Haskell, you want most of your code to be "pure" - without outside effects. But even things like printing to the terminal are considered side effects. These actions require an "IO" annotation on your function. But a lot of other operations need this as well. In this module, we'll explore a number of useful and fundamental operations that require the "IO Monad".
Module 5: Monads and Functional Structures
Having learned about the IO Monad in module 4, it's a good idea to understand the concept of monads more completely. We'll start with some simpler abstract structures, like Functors and Applicative functors. Then we'll explore the most common monads you might use, and see some reasons why they are useful!
Module 6: String and Numeric Types
Haskell's type system has a lot of advantages in helping us design our programs. But there are also some drawbacks. For various reasons, there are half a dozen different string types and twice as many numeric types!
It can be very frustrating when you are trying to use a library function, but you get stuck because it uses a different string type than you have in your program. This module will teach you how to manage these differences. In module 6, you'll learn about the different numeric types, the different string types, and how to convert between them.
Module 7: Solving Functional Problems
Programming ultimately requires solving problems. And in Haskell, your approach to problem solving is often a bit different than in other languages. In this module, we'll go over a number of different solution approaches to some common functional problems, so you have more confidence in approaching real world problems!
Final Project
The ultimate goal of this course is for you to feel confident enough in your Haskell to write a small project. Exercises help you learn syntax, but they aren't the same as project-based learning. To give you some more practice working in a project, there is a "Final Project" where you can build a code generator. With your program, you'll be able to specify a "type" in a YAML definition and quickly produce code for that type in 3 different languages!
Special Bonus: Making Sense of Monads
Module 5 gives an introductory view of Functional Structures like functors, applicative functors, and monads. But later on I decided this subject deserved its own course, so I adapted a lot of that material as "Making Sense of Monads" Since a lot of the material is similar, I decided to bundle the new course together with Haskell From Scratch. You can read more about it on the Making Sense of Monads page!
You can keep reading below for a lecture-by-lecture outline and FAQ!
Your Instructor
James is a Software Engineer living in Atlanta, GA. He first discovered Haskell in college, but began using it consistently since 2015. His Haskell experience blends multiple side projects as well as professional work. In late 2016, he began the Monday Morning Haskell blog, where he publishes new Haskell content every week.
Course Curriculum
-
Start1-1: Course Introduction (3:17)
-
Preview1-2: Basic Types (2:56)
-
Preview1-3: Compound Types (3:37)
-
Start1-4: Functions and Operators (6:08)
-
Start1-5: Writing Haskell Files (3:49)
-
Start1-6: Beyond Basic Syntax (10:05)
-
Start1-7: Anonymous Functions and Operators (3:58)
-
Start1-8: Purity, Immutability and Laziness (5:26)
-
Start1-9: Module 1 Review (1:39)
-
Start2-1: Creating Types (5:21)
-
Start2-2: Types and Newtypes (4:08)
-
Start2-3: Parameterized Types (2:13)
-
Start2-4: Building our Own Lists and Tuples (4:54)
-
Start2-5: Typeclasses I (4:19)
-
Start2-6: Typeclasses II (4:00)
-
Start2-7: Writing Our Own Typeclasses (2:55)
-
Start2-8: Polymorphic Data and Functions (3:56)
-
Start2-9: Module 2 Review (1:39)